Hi all, no bug report or feature request here, I just wanted to share some of my findings that I hope will be helpful to anyone who wants to achieve good performance when you have a game with hundreds of characters animating in a crowd.
I've recently converted my entire project of dozens of skeletons from SkeletonMecanim to SkeletonAnimation. I started to suspect that Mecanim had a CPU overhead that couldn't be justified. For context, my game is a spooky zoo management sim that needs to animate and render hundreds of zoo visitors wandering about, not to mention the player zookeeper, the staff of the zoo and the monsters themselves.
To confirm my hunch that SkeletonMecanim produced pretty poor performance when dealing with hundreds of skeletons in comparison to SkeletonAnimation, here's the test I made, first with SkeletonMecanim:
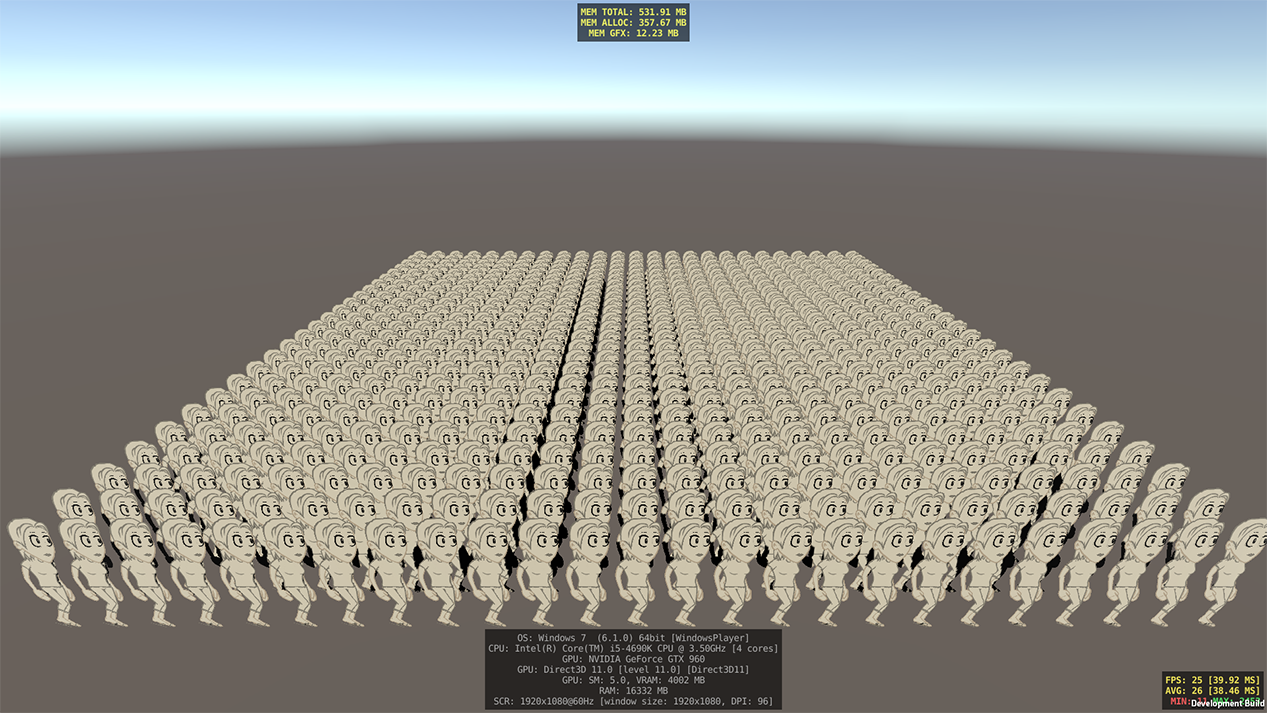
And then with SkeletonAnimation:
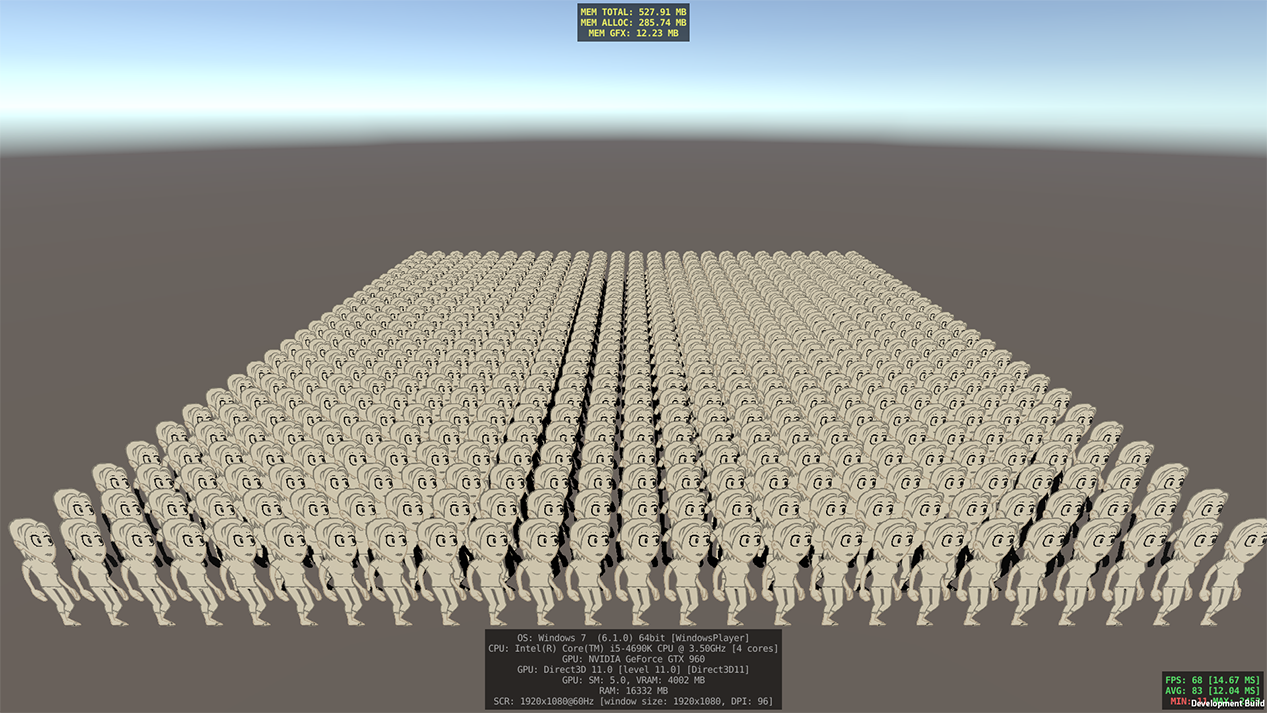
That's 625 visitor skeletons doing their walk-cycle in both tests. Yes, I know they look exactly the same, but trust me; the mode of driving the SkeletonRenderer is different! (This is with Unity 2020.1.9, by the way, with the latest Spine and URP shaders, and if you click the image above you can see my quite modest hardware listed at the bottom of the screen)
For reference, the skeleton I'm using is pretty simple:
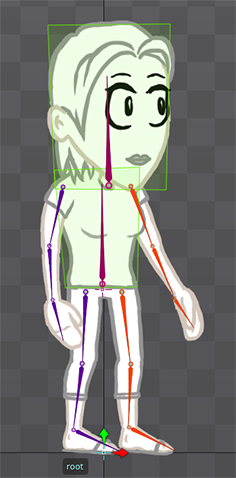
One not-so-simple aspect is the Animator Controller in Unity for the SkeletonMecanim characters, where there are 10 separate layers. That's a lot! And that's because I need to mix a lot of different animations on top of one another, affecting different areas of the body. If you don't have as many layers in your Animator Controller, your results may vary. With SkeletonAnimation, you just take an animation you want to mix 'on top of' another one by mixing it into a TrackEntry.
In any case, here are my results, courtesy of Unity's Profile Analyzer, which takes 300 frames from both tests and puts them side-by-side with helpful averages and direct comparisons:
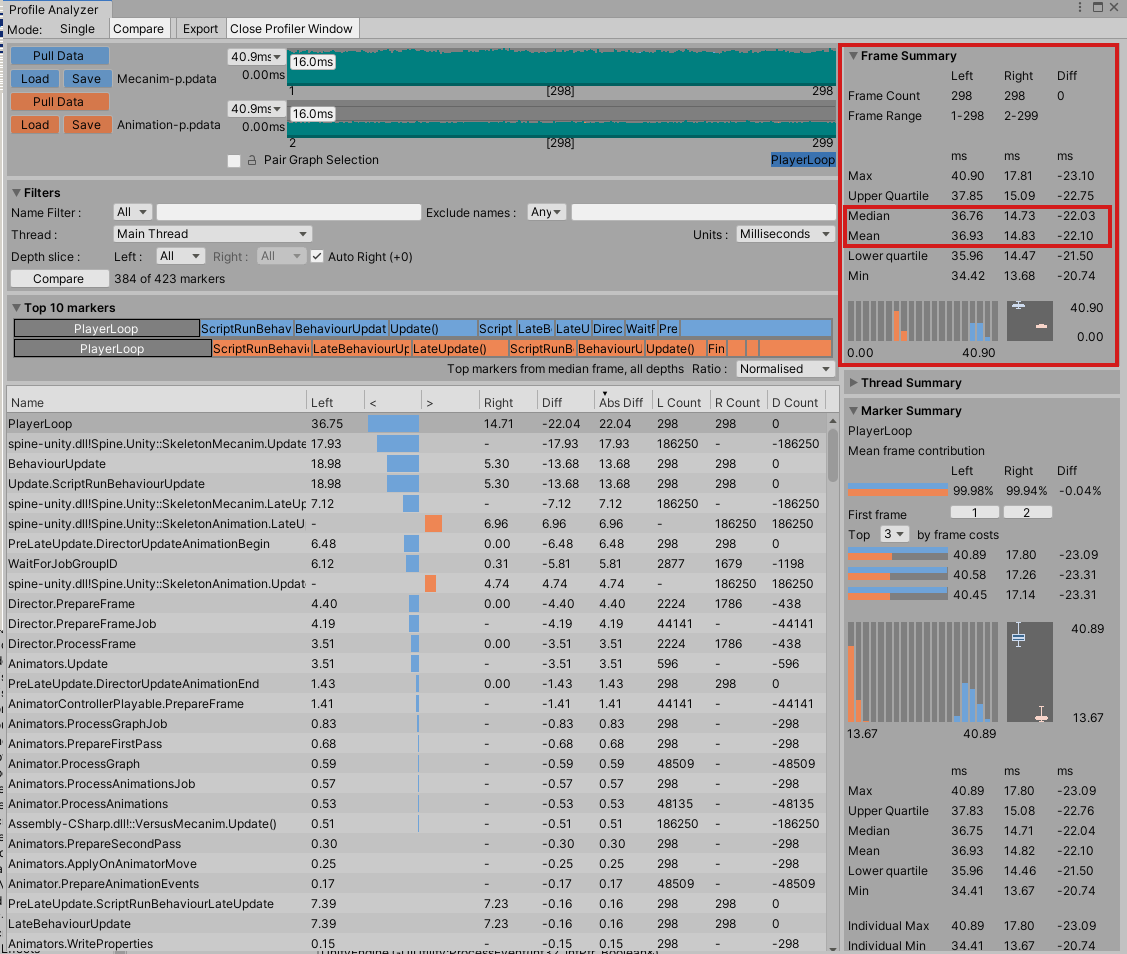
The short of it is that the mean average of the SkeletonMecanim test is 27 frames per second, and the mean average of the SkeletonAnimation test is 67 frames per second.
I knew that SkeletonMecanim was going to be slower and heavier, but I didn't imagine it would be more than twice as slow in my case. (I'm guessing that's the cost of my Animation Controller having too many layers.) A deeper dive into the individual markers on the left of the screenshot above shows that SkeletonMecanim.Update and LateUpdate are both more costly than SkeletonAnimation.Update and LateUpdate. You also have to put up with the cost of the 'Director' (Unity's Mecanim agent) running PreLateUpdate.DirectorUpdateAnimationBegin and Director.PrepareFrame and so on, and then Animators.ProcessWhatever too. There's just more overhead as Mecanim churns through it's tasks
After this test, I then immediately went and ripped Mecanim out of my project. While I miss the expressive way of describing how animations chain together which you get in Unity's Animator window, in the real world of my actual game project; it runs about 10 frames per second faster than it did before. Well worth trying this test, and the week of work it took to migrate.
Posting all this here in the hope that future programmers of games with crowds in them, won't bother with Mecanim at all - please do link to it when discussions of performance come up.